You can’t launch the nuke’s with out having two keys to turn, this post will look at the keys and buttons.<p>
My objective was to recreate to "two keys" experience, this would need two keys that have switches hooked to the Raspberry Pi, the locks need to be quite good quality as I did not want anyone picking them.
The choice was to go with Abloy Protec2. They are very hard to pick,and they look the part.
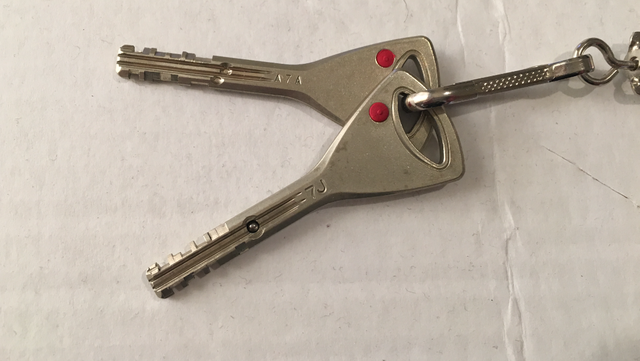
Security Snobs seemed to have the best price for protec2 locks, the locks with the switch are quite a bit more expensive and so I went with furniture locks and added my own micro-switches.
Keys are all good, but the players will want to play with the console before they get the keys, so we invented a test mode, with key override buttons.
The first version of the wiring was to have a 3.3V wire and the input wire, then setup the switch across the two wires.
This seems like the obvious solution, but this caused nothing but stray inputs. A quick session of swear words and Google told me that the fix was to use pull down resistors on the input lines.
Raspberry Pi even lets you do this in software, you just add "pull_up_down=GPIO.PUD_DOWN" when you setup the input, that means that the un-connected pin is puched low and not left floating.
This did improve things a bit but still getting stray inputs about 1 in 10 times so another session of swear words and it was decided to use a full three wire system. The input line is switch either high or low at all times and never left to float.
The code for the keys / buttons needs to be able to trigger if the keys are turned within 200ms of each other, but we don't know which one will be first.
The code looks like this:
def SetLaunchKeyA(inputpin): global key_SW1 global key_SW2 if(GPIO.input(33)): key_SW1=time.time() else: key_SW1=0 if (GPIO.input(33))and(GPIO.input(37)): if (abs(key_SW1 - key_SW2) <0.2): Boom() def SetLaunchKeyB(inputpin): global key_SW1 global key_SW2 if(GPIO.input(37)): key_SW2=time.time() else: key_SW2=0 if (GPIO.input(33))and(GPIO.input(37)): if (abs(key_SW1 - key_SW2) <0.2): Boom()This is called by an IO interrupt, when a key event happens, we check if the input is high or low, if high then set key_SW1 to current time if it is low then we set key_SW1 to 0.
If both keys set high, then we can check the time difference.
This is the internal wiring, simple and ugly
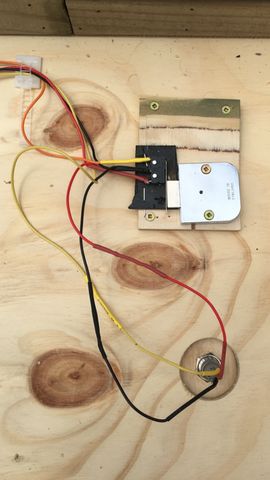